Guided in-process fuzzing of Chrome components
August 5th, 2016 | by Google Security PR | published in Google Online Security
Posted by Max Moroz, Chrome Security Engineer and Kostya Serebryany, Sanitizer Tsar
In the past, we’ve posted about innovations in fuzzing, a software testing technique used to discover coding errors and security vulnerabilities. The topics have included AddressSanitizer, ClusterFuzz, SyzyASAN, ThreadSanitizer and others.
Today we’d like to talk about libFuzzer (part of the LLVM project), an engine for in-process, coverage-guided, white-box fuzzing:
- By in-process, we mean that we don’t launch a new process for every test case, and that we mutate inputs directly in memory.
- By coverage-guided, we mean that we measure code coverage for every input, and accumulate test cases that increase overall coverage.
- By white-box, we mean that we use compile-time instrumentation of the source code.
Sample Fuzzer
extern “C“ int LLVMFuzzerTestOneInput(const uint8_t* data, size_t size) {
std::string buf;
woff2::WOFF2StringOut out(&buf);
out.SetMaxSize(30 * 1024 * 1024);
woff2::ConvertWOFF2ToTTF(data, size, &out);
return 0;
}
See also the build rule.
Sample Bug
#5 0x4dbfd6 in LLVMFuzzerTestOneInput testing/libfuzzer/fuzzers/convert_woff2ttf_fuzzer.cc:15:3
Check out our documentation for additional information.
Integrating LibFuzzer with ClusterFuzz
ClusterFuzz is Chromium’s infrastructure for large scale fuzzing. It automates crash detection, report deduplication, test minimization, and other tasks. Once you commit a fuzz target into the Chromium codebase (examples), ClusterFuzz will automatically pick it up and fuzz it with libFuzzer and AFL.
ClusterFuzz supports most of the libFuzzer features like dictionaries, seed corpus and custom options for different fuzzers. Check out our Efficient Fuzzer Guide to learn how to use them.
Besides the initial seed corpus, we store, minimize, and synchronize the corpora for every fuzzer and across all bots. This allows us to continuously increase code coverage over time and find interesting bugs along the way.
ClusterFuzz uses the following memory debugging tools with libFuzzer-based fuzzers:
- AddressSanitizer (ASan): 500 GCE VMs
- MemorySanitizer (MSan): 100 GCE VMs
- UndefinedBehaviorSanitizer (UBSan): 100 GCE VMs
Sample Fuzzer Statistics
It’s important to track and analyze performance of fuzzers. So, we have this dashboard to track fuzzer statistics, that is accessible to all chromium developers:
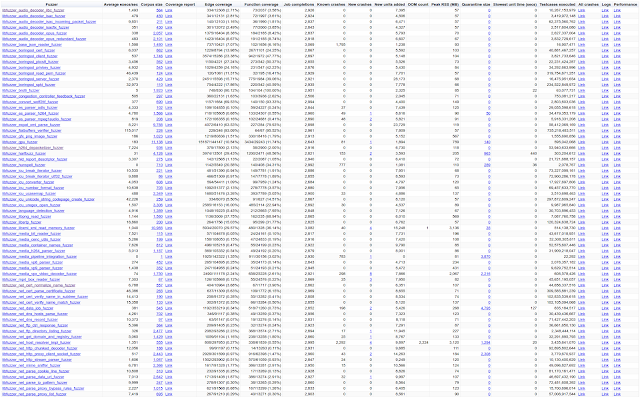
- 120 fuzzers
- 112 bugs filed
- Aaaaaand…. 14,366,371,459,772 unique test inputs!
Analysis of the bugs found so far
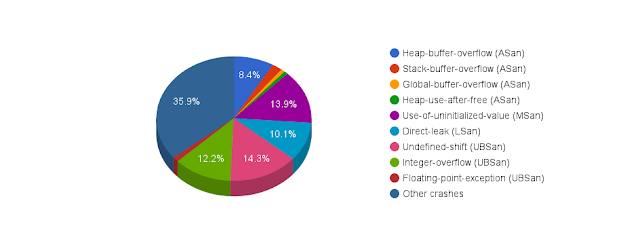