Geolocation and Firebase for the Internet of Things
December 21st, 2016 | by Maps Devel | published in Google Maps
Posted by Ken Nevarez, Industry Solutions Lead at Google
GPS is the workhorse of location based services, but there are use cases where you may want to avoid the cost and power consumption of GPS hardware or locate devices in places where GPS lacks accuracy, such as in urban environments or buildings.
We’ve seen recent growth in Internet of Things (IoT) applications using the Google Maps Geolocation API instead of GPS for asset tracking, theft prevention, usage optimization, asset servicing, and more. As part of my 20 percent project at Industry Solutions, I created a prototype IoT device that can locate itself using surrounding WiFi networks and the Google Maps Geolocation API. In this post, I’ll discuss some interesting implementation features and outline how you can create the prototype yourself.
I built a device that scans for local WiFi and writes results (WiFi hotspots and their signal strength) to a Firebase Realtime Database. A back-end service then reads this data and uses the Google Maps Geolocation API to turn this into a real-world location, which can be plotted on a map.
Set up the Device & Write Locally
For this proof of concept, I used the Intel Edison as a Linux-based computing platform and augmented it with Sparkfun’s Edison Blocks. To build the device, you will need an Intel Edison, a Base Block, a Battery Block and a Hardware pack.
Developing for the Edison is straightforward using the Intel XDK IDE. We will be creating a simple Node.js application in JavaScript. I relied on 3 libraries: Firebase for the database connection, wireless-tools/iwlist to capture WiFi networks, and macaddress to capture the device MAC. Installation instructions can be found on the linked pages.
Step 1: get the device MAC address and connect to Firebase:
function initialize() { macaddress.one('wlan0', function (err, mac) { mac_address = mac; if (mac === null) { console.log('exiting due to null mac Address'); process.exit(1); } firebase.initializeApp({ serviceAccount: '/node_app_slot/.json', databaseURL: 'https:// .firebaseio.com/' }); var db = firebase.database(); ref_samples = db.ref('/samples'); locationSample(); }); }
The above code contains two placeholders:
- The service-account-key is a private key you create in the Firebase Console. Follow the gear icon in the upper left of console, select “settings”, and click Generate New Private Key. Place this key on your Edison in the directory /node_app_slot/. See this Firebase documentation for more information.
- The project-id in the database URL is found in the Firebase console database page after you have linked your Google project with Firebase.
Step 2: scan for WiFi networks every 10 seconds and write locally:
function locationSample() { var t = new Date(); iwlist.scan('wlan0', function(err, networks) { if(err === null) { ref_samples.push({ mac: mac_address, t_usec: t.getTime(), t_locale_string: t.toLocaleString(), networks: networks, }); } else { console.log(err); } }); setTimeout(locationSample, 10000); }
Write to the cloud
The locationSample() function above writes detectable WiFi networks to a Firebase database that syncs to the cloud when connected to a network.
Caveat: To configure access rights and authentication to Firebase, I set up the device as a “server”. Instructions for this configuration are on the Firebase website. For this proof of concept, I made the assumption that the device was secure enough to house our credentials. If this is not the case for your implementation you should instead follow the instructions for setting up the client JavaScript SDK.
The database uses 3 queues to manage workload: a WiFi samples queue, a geolocation results queue and a visualization data queue. The workflow will be: samples from the device go into a samples queue, which gets consumed to produce geolocations that are put into a geolocations queue. Geolocations are consumed and formatted for presentation, organized by device, and the output is stored in a visualizations bucket for use by our front end website.
Below is an example of a sample, a geolocation, and our visualization data written by the device and seen in the Firebase Database Console.
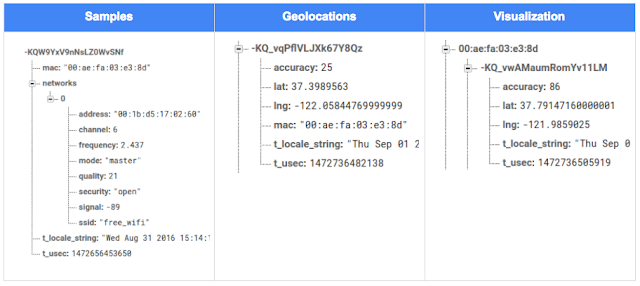
Processing the Data with Google App Engine
To execute the processing of the sample data I used a long running Google App Engine Backend Module and a custom version of the Java Client for Google Maps Services.
Caveat: To use Firebase with App Engine, you must use manual scaling. Firebase uses background threads to listen for changes and App Engine only allows long-lived background threads on manually scaled backend instances.
The Java Client for Google Maps Services takes care of a lot of the communications code required to use the Maps APIs and follows our published best practices for error handling and retry strategies that respect rate limits. The GeolocateWifiSample() function below is registered as an event listener with Firebase. It loops over each network reported by the device and incorporates it into the geolocation request.
private void GeolocateWifiSample(DataSnapshot sample, Firebase db_geolocations, Firebase db_errors) { // initalize the context and request GeoApiContext context = new GeoApiContext(new GaeRequestHandler()).setApiKey(""); GeolocationApiRequest request = GeolocationApi.newRequest(context) .ConsiderIp(false); // for every network that was reported in this sample... for (DataSnapshot wap : sample.child("networks").getChildren()) { // extract the network data from the database so it’s easier to work with String wapMac = wap.child("address").getValue(String.class); int wapSignalToNoise = wap.child("quality").getValue(int.class); int wapStrength = wap.child("signal").getValue(int.class); // include this network in our request request.AddWifiAccessPoint(new WifiAccessPoint.WifiAccessPointBuilder() .MacAddress(wapMac) .SignalStrength(wapStrength) .SignalToNoiseRatio(wapSignalToNoise) .createWifiAccessPoint()); } ... try { // call the api GeolocationResult result = request.CreatePayload().await(); ... // write results to the database and remove the original sample } catch (final NotFoundException e) { ... } catch (final Throwable e) { ... } }
Register the GeolocateWifiSample() function as an event handler. The other listeners that process geolocation results and create the visualization data are built in a similar pattern.
ChildEventListener samplesListener = new ChildEventListener() { @Override public void onChildAdded(DataSnapshot dataSnapshot, String previousChildName) { // geolocate and write to new location GeolocateWifiSample(dataSnapshot, db_geolocations, db_errors); } ... }; db_samples.addChildEventListener(samplesListener);
Visualize the Data
To visualize the device locations I used Google App Engine to serve stored data from Firebase and the Google Maps JavaScript API to create a simple web page that displays the results. The index.html page contains an empty
function initMap() { // attach listeners firebase.database().ref('/visualization').on('child_added', function(data) { ... data.ref.on('child_added', function(vizData) { circles[vizData.key]= new CircleRoyale(map, vizData.val().lat, vizData.val().lng, vizData.val().accuracy, color); set_latest_position(data.key, vizData.val().lat, vizData.val().lng); }); data.ref.on('child_removed', function(data) { circles[data.key].removeFromMap(); }); }); // create the map map = new google.maps.Map(document.getElementById('map'), { center: get_next_device(), zoom: 20, scaleControl: true, }); ... }
Since the API returns not only a location but also an indication of accuracy, I’ve created a custom marker that has a pulsing radius to indicate the accuracy component.
Two devices (red and blue) and their last five known positions |
What’s next?
In this post I’ve outlined how you can build an IoT device that uses Google Maps Geolocation API to track any internet-connected device – from robotics to wearables. The App Engine processing module can be expanded to use other Google Maps APIs Web Services providing geographic data such as directions, elevation, place or time zone information. Happy building!
As an alternative, you can achieve a similar solution using Google Cloud Platform as a replacement for Firebase—this article shows you how.
|
About Ken: Ken is a Lead on the Industry Solutions team. He works with customers to bring innovative solutions to market. |
Engage holiday shoppers with Promoted Places in Google Maps
December 15th, 2016 | by Rob Newton | published in Google Adwords, Google Maps
During the busy holiday season, on-the-go shoppers are using their smartphones to find the perfect stocking stuffers, festive decor and gifts for loved ones. In fact, 70% of smartphone users who bought something in a store first turned to their devices for information relevant to that purchase. One in four of them also used a map through a web browser or an app before making the purchase.1 This year, a number of brands are testing Promoted Places in Google Maps to showcase special offers and announcements to drive more foot traffic to their stores.
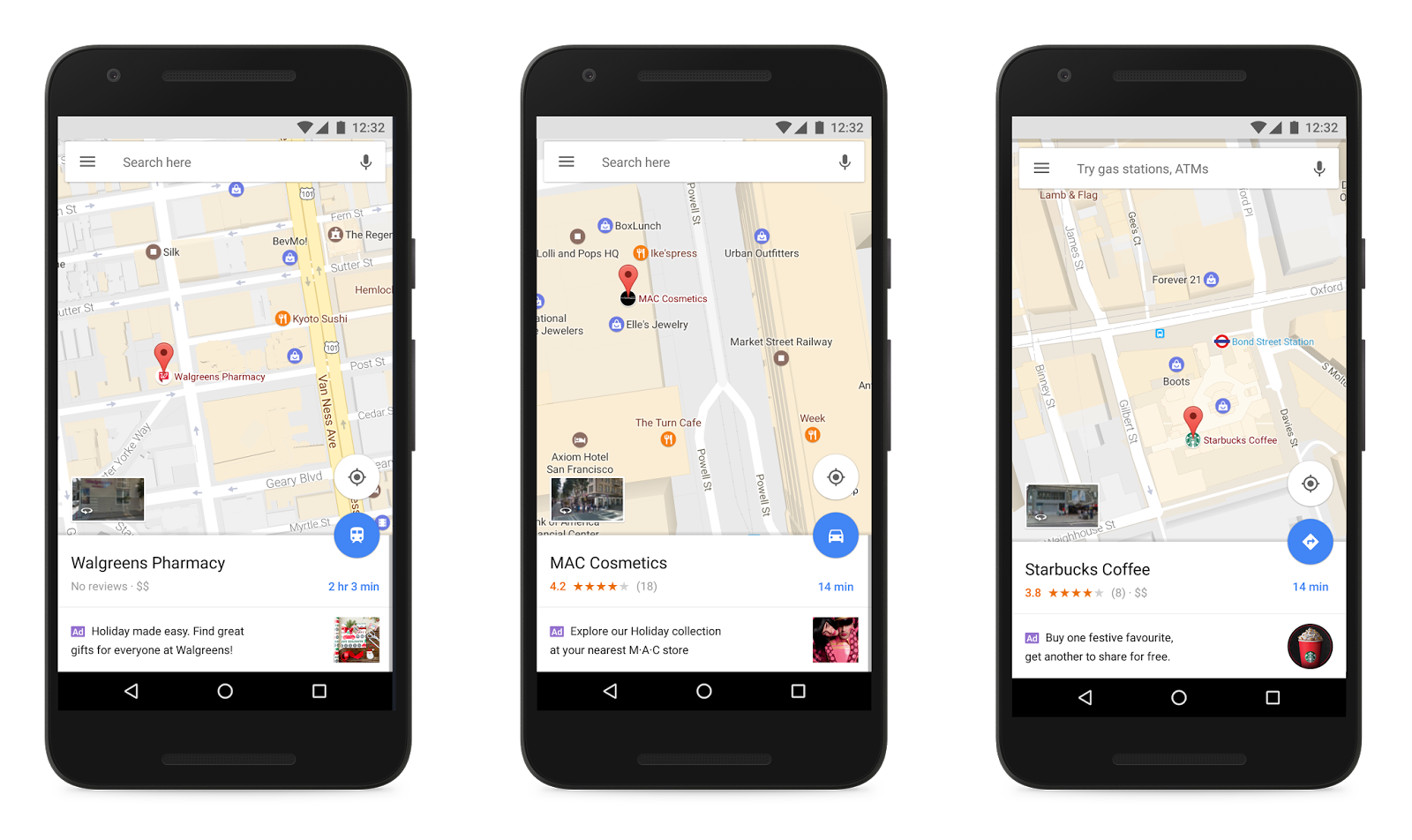
Some users have started seeing a limited test of Promoted Places in the Google Maps for Android app. It integrates seamlessly with the browsing experience, where places of interest are visually marked with icons to help users orient themselves and explore the world around them. With Promoted Places, advertisers can improve awareness for their stores by branding their location icons with their logo. Clicks on a business’s logo will reveal promotions such as in-store sales or new products and services to help consumers decide where to go. They can also view the business’s place page to see store hours, check live updates to Popular Times, get directions, and more.
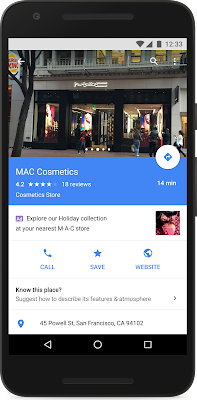
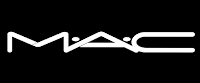
“We’re looking forward to testing Promoted Places to help us deliver a more branded and customized experience on Google Maps. It will allow us to spotlight our latest products with the goal of driving more shoppers in-store to get the full M·A·C Cosmetics experience.”
- Laura Elkins, SVP of Global Marketing
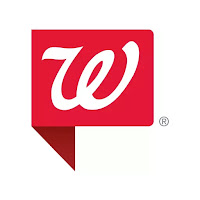
“Innovation is part of our DNA and we’re continually evaluating meaningful and contextually relevant ways to connect with our customers. Staying top of mind, especially during the competitive holiday season, requires a mix of tried and true, including breakthrough strategies. Promoted Places in Google Maps can help drive incremental store traffic and promote Walgreens as a go-to destination for small gifts. This gives us a new and different way to highlight seasonal offerings through visuals and engaging copy. Combined with the breadth of our store locations in the community, this aligns with our goals of driving loyalty and giving customers more reasons to choose Walgreens for their holiday needs.”
- Andrea Kaduk, Director of SEM & Social
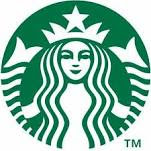
“With Promoted Places, we can enhance our presence on Google Maps and give users a glimpse of our brand and coffeehouses before they even get there. Having our logo on the map helps our locations jump out and promoting our favourite menu items gives people a reason to choose us.”
- Jamie McQuary, Senior Marketing Manager
This holiday season, Promoted Places will help businesses stand out to busy shoppers in the moments they’re figuring out where to go. We’re excited to test new experiences that help consumers discover nearby sales and promotions and allow businesses to reach more people through Google Maps.
Posted by: Cynthia Johanson, Product Manager, AdWords
1. Google/Purchased Digital Diary: How Consumers Solve Their Needs in the Moment, May 2016, Smartphone users = 1000. Offline Purchasers = 696
Address Geocoding in the Google Maps APIs
November 22nd, 2016 | by Maps Devel | published in Google Maps
Forward Geocoding is the process of converting addresses (like a street address) into geographic coordinates (latitude and longitude), which you can use to place markers on a map or position the map. The Google Maps APIs have several services that you can use to convert addresses into coordinates – the Geocoding API, the Place Autocomplete service in Places API, and the Place Search service in Places API. What are the differences between them and when should you use each one? Here’s where to start.
Note that while this blog focuses on the server-side Places and Geocoding APIs, these best practices also apply to the client-side Places and Geocoding services in the Google Maps JavaScript API.
Geocoding API
The Geocoding API is best for handling unambiguous queries: complete postal address strings (for example, “48 Pirrama Rd, Pyrmont, NSW, Australia”). Compared to other Google APIs, the Geocoding API provides the best quality matching of addresses globally for these types of complete, unambiguous queries. However, Geocoding API is not recommended if your application handles ambiguous or incomplete queries, such as “123 Main St”, or if it handles queries that may contain non-address information such as apartment numbers or business names.
Geocoding API is best used for unambiguous complete addresses, such as “48 Pirrama Rd, Pyrmont, NSW, Australia” |
Places API
The Places API allows users to discover both addresses and semantic locations, such as cafes or parks, by name or type. In contrast to the Geocoding API, it handles ambiguous or incomplete requests in a more robust way. If your application handles user interaction, or addresses that are ambiguous or incomplete, consider the following services.
Place Autocomplete service
For applications that respond in real time to user input, we recommend using the Place Autocomplete service in the Places API. This service is designed to return multiple possible addresses and allow the user to choose between them. The autocomplete lookup function can also be biased to return results specific to a location, enabling high quality results to be returned for incomplete queries such as “123 Main St”. Since the Place Autocomplete service is optimized for responding to user input, it also has very low latency, usually at least 10x lower than the Geocoding API. It’s also good at handling misspelled queries, or queries containing non-address information, since as the user types, they can see suggestions and correct their spelling if needed.
Typing “123 Main St” into a Place Autocomplete search box lets the user choose from multiple results. Results can also be biased to prefer those near the area shown on the map or near the current user location |
Place Search service
The Place Autocomplete service relies on a user to choose the best option from multiple results. What if you have an application that handles ambiguous or incomplete queries in an automated fashion, with no user able to provide input?
For geocoding ambiguous or incomplete addresses in automated systems, when there is no user to select one of the autocomplete suggestions, we recommend the Place Search service in Places API. Place Search is better at coping with ambiguous queries than the Geocoding API, and lets you restrict your search to a specified area, or rank results by distance, allowing more precise filtering and ranking of results for ambiguous or incomplete queries. Place search is also more robust at responding to queries with additional non-address information such as business names or apartment numbers.
Future Changes to Geocoding API
We plan to roll out an update to the Geocoding API at the end of November 2016 that will increase the difference between Geocoding and Places performance for ambiguous and unambiguous queries. This change will improve the quality of Geocoding results for unambiguous queries, but will be more likely to return ZERO_RESULTS for ambiguous or incomplete queries where the Geocoding API was unable to find a high quality result.
If you are already using the above best practices, you should see an improvement in your Geocoding API results. If you are currently using the Geocoding API for incomplete or ambiguous queries, or for queries that may contain non-address information such as business names or apartment numbers, we recommend that you switch to the Places API instead, as it is likely to give better quality results for your use case.
You can try the new Geocoding service ahead of launch by adding an optional parameter, new_forward_geocoder=true, to your Geocoding API request. For example:
https://maps.googleapis.com/maps/api/geocode/json?new_forward_geocoder=true&address=1600+Amphitheatre+Parkway,+Mountain+View,+CA&key=YOUR_API_KEY
If you want to try the new Geocoding service in the JavaScript Maps API Geocoding Service, you can set the new optional parameter newForwardGeocoder: true in the GeocoderRequest object. The new Geocoding service will launch for both the Geocoding API and the Geocoding Service in the JavaScript Maps API at the same time. All of the recommendations in this blog post apply to both the server-side and client-side APIs.
If you have any bug reports or feature requests for the new Geocoding service, please let us know using our public issue tracker.
In Summary
The following table sums up when we recommend you use the Geocoding API, Place Autocomplete service and Place Search service.
Geocoding API | Place Search | Place Autocomplete | |
---|---|---|---|
Scope | Addresses only | Semantic locations and addresses, including businesses and points of interest | |
Strengths | Unambiguous complete addresses | Ambiguous or incomplete addresses in automated systems | Responding to real-time user input |
If your application does not yet follow these best practices, you may get worse results from Geocoding API in future, so we recommend you test how your application works with the new Geocoding service and update your application to use the above best practices if required. Try the upcoming Geocoding service by setting new_forward_geocoder=true in your geocoding request.
For more information on the Google Maps Geocoding API, Place Autocomplete in the Places API and Place Search in the Places API, please see the developer documentation. Also see this more detailed best practices guide in our documentation for more details on Geocoding best practices for various use cases, including minimizing latency when querying Directions API with addresses.
Finally, a big thank you to all the developers who use the Google Maps Geocoding API and provide feedback via the issue tracker. Getting feedback from developers is vital for us to be able to keep improving our products, so if you have any bug reports or feature requests, please let us know!
|
Posted by Elena Kelareva, Product Manager, Google Maps APIs |
Smart scrolling comes to mobile web maps
November 15th, 2016 | by Maps Devel | published in Google Maps
If you’re building a website today, your users are more likely to view it on a mobile device than on a desktop or laptop. Google has plenty of resources to help developers make their websites stand out on mobile, from a guide to building mobile-friendly websites, to a mobile-friendly testing tool, to promoting new mobile web technologies such as Accelerated Mobile Pages and Progressive Web Apps.
Mobile web users often get frustrated when trying to scroll the page, but an embedded map captures their swipe and pans the map instead. This can even lead to users getting stuck on the map and having to reload the page in order to get back to the rest of the page.
Today we’re introducing intuitive scrolling for sites with embedded maps and making the full-screen control visible by default on mobile devices. This should give your users a more intuitive and less frustrating map interaction experience on mobile browsers.
The map trap
We have added a new gestureHandling option to the Google Maps JavaScript API. This setting controls how touch gestures* on the map are handled.
Values:
- “cooperative”: Two-finger touch gestures pan and zoom the map, as do all mouse gestures. One-finger touch gestures are ignored by the map. In this mode, the map cooperates with the page, so that one-finger touch gestures can pan the page.
- “greedy”: All touch gestures pan or zoom the map. This was the previous behaviour.
- “none”: The map cannot be panned or zoomed by user gestures.
- “auto”: Gesture handling is automatically set to either cooperative or greedy, depending on whether the page is scrollable or not (defined by a comparison of the page body dimensions and the window dimensions).
- If the page is scrollable, “auto” sets the gesture handling mode to cooperative.
- If the page is not scrollable, “auto” sets the gesture handling to greedy.
- If the map is in an iFrame, “auto” sets the gesture handling to cooperative because the API can’t determine whether the page is scrollable.
*Note that there is currently no way to change the gesture handling mode for Street View; these options only affect the way gestures are handled by the map. If you’d like to see this extended to Street View in future, please let us know on our public issue tracker.
You can enable any of these four gesture handling modes by adding the corresponding field to the MapOptions object. For example:
map = new google.maps.Map(document.getElementById(‘map-div’), {
gestureHandling: ‘cooperative’,
center: {lat: -34.397, lng: 150.644},
zoom: 8
});
If the gestureHandling option is not set, the default value is auto, since that automatically chooses what we expect to be the best behavior based on what the browser can detect about the placement of your map in the page. If you prefer to always use the old map gesture handling mode for users viewing your site on mobile devices, change the value of gestureHandling to greedy, which sends all user gestures to the map.
![]() |
Maps viewed within a scrollable website on a mobile device will display this overlay on touch |
The option draggable: false has now been superseded by gestureHandling: ‘none’. The old option draggable is now deprecated, but we’ll maintain backwards compatibility. Developers who previously turned off map interaction by setting draggable to false will keep their existing non-interactive maps.
Maximizing the map when you need it
Many users in our user studies said they found small embedded maps on mobile devices hard to interact with and they preferred to interact with a larger map. To address this request, we’ve made the fullscreen control visible by default on mobile devices. The fullscreen control allows the user to make the map larger. When the map is in fullscreen mode, the user can pan the map using one finger. As a developer, you can enable or disable fullscreen control, by setting the fullscreenControl option to true or false in the MapOptions object. When the map is in fullscreen mode, one finger will always pan the map, since there is no surrounding page to pan.
The default setting of fullscreenControl is true on mobile browsers, and false on desktop browsers, since the problem of maps being too small for interaction usually only occurs on mobile devices.
The fullscreen control allows the user to make the map larger for easier interaction |
View this demo on a mobile device to see how the fullscreen button and cooperative gesture handling mode (or auto gesture handling mode on a scrollable site) will look to your users.
For more information on the Google Maps JavaScript API, please see the developer documentation or review the latest release notes.
A big thank you to all the developers who use the Google Maps JavaScript API and provide feedback via the issue tracker. Getting feedback from developers is vital for us to be able to keep improving our products, so if you have any bug reports or feature requests, please let us know.
|
Posted by Elena Kelareva, Product Manager, Google Maps APIs |
Key Improvements for Your Maps API Experience
October 4th, 2016 | by Maps Devel | published in Google Maps
Originally posted on the Google Developers blog.
Posted by Israel Shalom, Product Manager
Here at Google, we’re serving more than a hundred APIs to ensure that developers have the resources to build amazing experiences with them. We provide a reliable infrastructure and make it as simple as possible so developers can focus on building the future. With this in mind, we’re introducing a few improvements for the API experience: more flexible keys, a streamlined ‘getting-started’ experience, and easy monitoring.
Faster, more flexible key generation
Keys are a standard way for APIs to identify callers, and one of the very first steps in interacting with a Google API. Tens of thousands of keys are created every day for Google APIs, so we’re making this step simpler — reducing the old multi-step process with a single click:
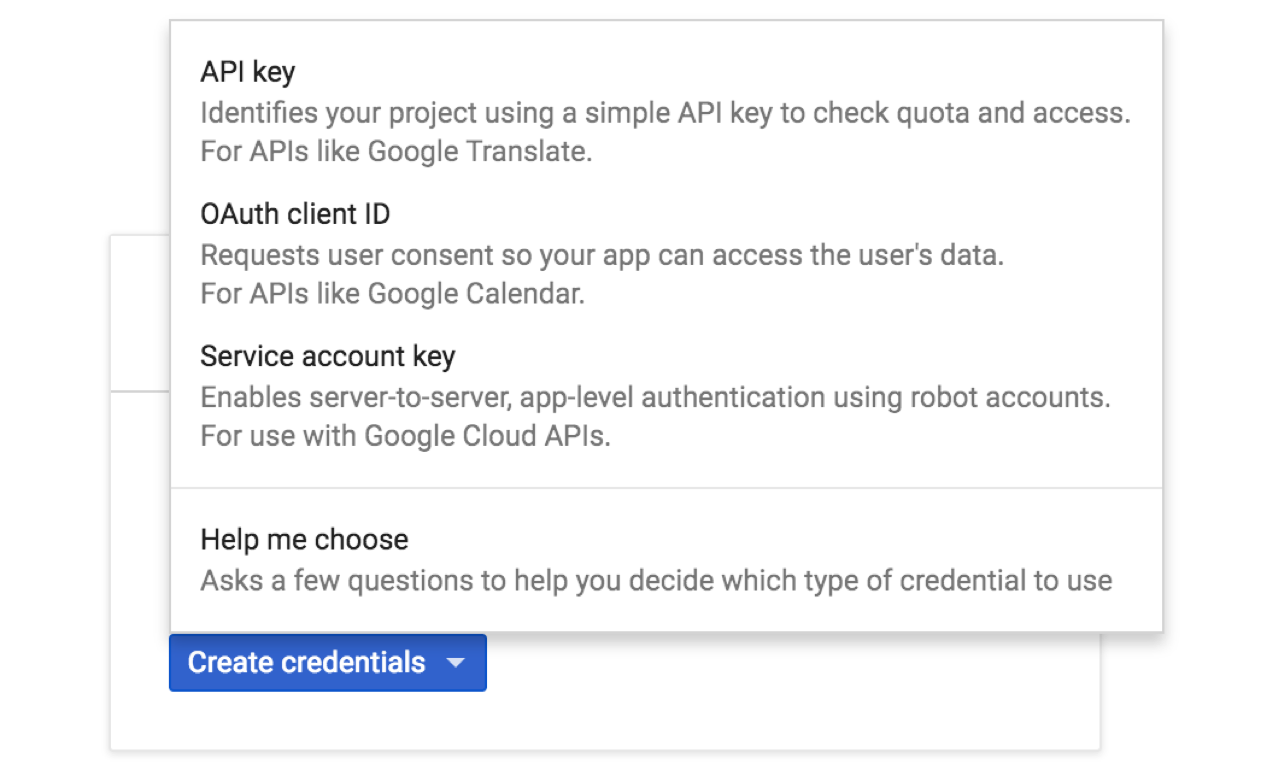
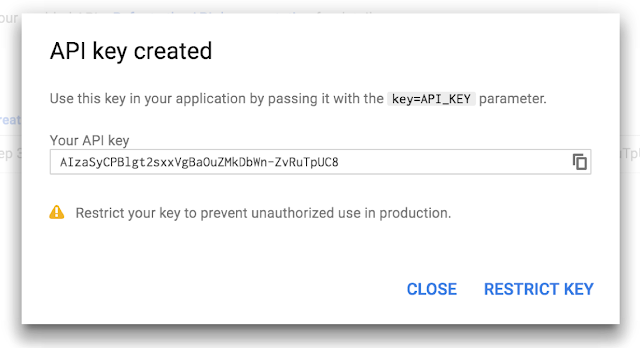
You no longer need to choose your platform and various other restrictions at the time of creation, but we still encourage scope management as a best practice:
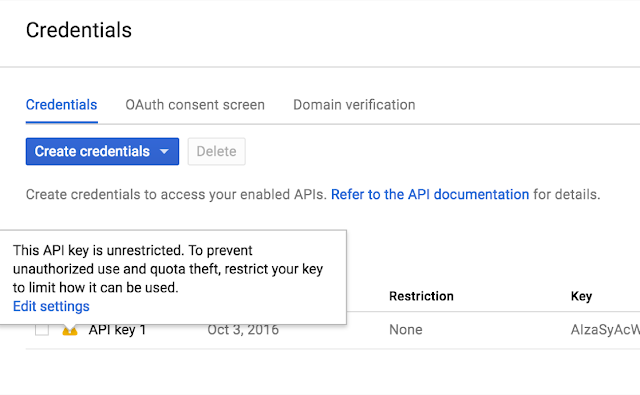
Streamlined getting started flow
We realize that many developers want to get straight to creation and don’t necessarily want to step into the console. We’ve just introduced an in-flow credential set up procedure directly embedded within the developer documentation:
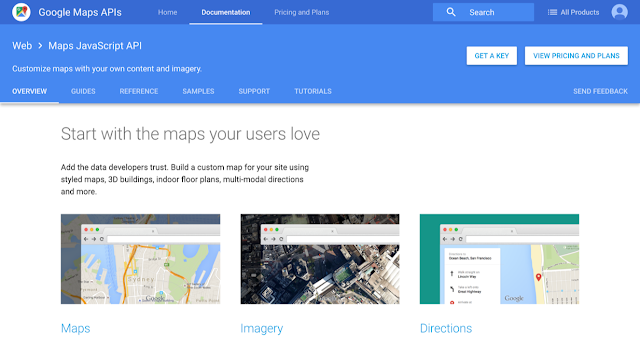
Click the ‘Get a Key’ button, choose or create a project, and then let us take care of enabling the API and creating a key.
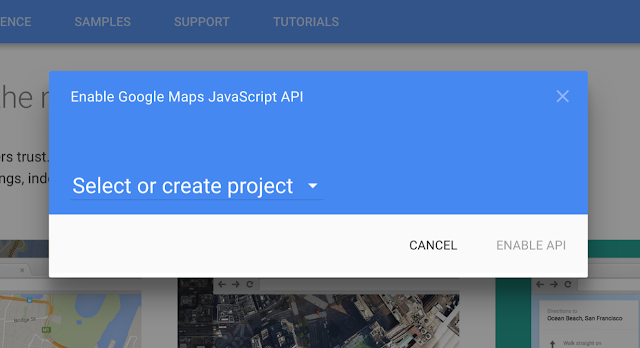
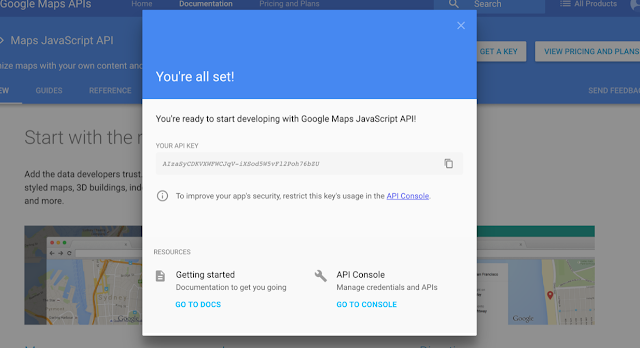
We are currently rolling this out for the Google Maps APIs and over the next few months we’ll bring it to the rest of our documentation.
API Dashboard
We’re not just making it easier to get started, we’re simplifying the on-going usage experience, too. For developers who use one or more APIs frequently, we’ve built the new API Dashboard to easily view usage and quotas.
If you’ve enabled any APIs, the dashboard is front and center in the API Console. There you can view all the APIs you’re using along with usage, error and latency data:
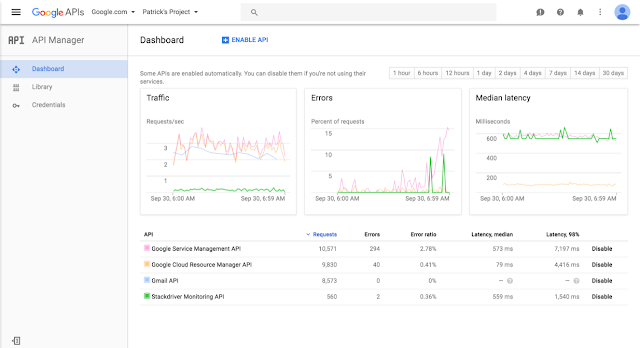
Clicking on an API will jump to a detailed report, where you’ll see the traffic sliced by methods, credentials, versions and response code (available on select APIs):
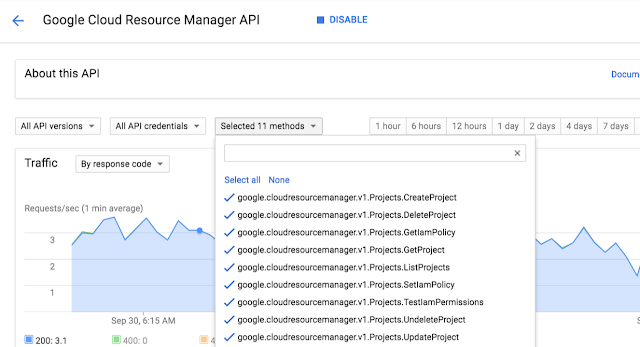
We hope these new features make your API usage easier, and we can’t wait to see what you’re going to build next!
A sizzling open source release for the Australian Election site
September 27th, 2016 | by Maps Devel | published in Google Maps
One of the best parts of my job at Google is 20 percent time. While I was hired to help developers use Google’s APIs, I value the time I’m afforded to be a student myself—to learn new technologies and solve real-world problems. A few weeks prior to the recent Australian election an opportunity presented itself. A small team in Sydney set their sights on helping the 15 million voters stay informed of how to participate, track real-time results, and (of course) find the closest election sausage sizzle!
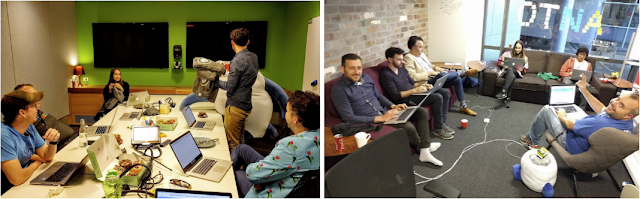
Our team of designers, engineers and product managers didn’t have an immediate sense of how to attack the problem. What we did have was the power of Google’s APIs, programming languages, and Cloud hosting with Firebase and Google Cloud Platform.
The result is a mish-mash of some technologies we’d been wanting to learn more about. We’re open sourcing the ausvotes.withgoogle.com repository to give developers a sense of what happens when you get a handful of engineers in a room with a clear goal and a immovable deadline.
The Election AU 2016 repository uses:
- Go from Google App Engine instances to serve the appropriate level of detail for users’ viewport queries from memory at very low latency, and
- Dart to render the live result maps on top of Google Maps JavaScript API using Firebase real time database updates.
A product is only as good as the attention and usage is receives. Our team was really happy with the results of our work:
- 406,000 people used our maps, including 217,000 on election day.
- We had 139 stories in the media.
- Our map was also embedded in major news websites, such as Sky News.
Complete setup and installation instructions are available in the Github README.
|
Posted by Brett Morgan, Developer Programs Engineer |
Custom map styling with the Google Maps APIs on Android and iOS
September 21st, 2016 | by Maps Devel | published in Google Maps
Your app, your map style. For iOS and Android. |
Cross-platform custom map styling is here—change the color palette of your maps, hide labels, vary road density and toggle points of interest. Your maps can now match your brand and style across your website and your apps!
The Google Maps APIs now support you in creating beautiful styled maps for your Android and iOS apps as well as your website using the same JSON style object.
Easily create your style
The new Google Maps APIs Styling Wizard helps you to create a map style in a few clicks. Use one of our pre-built styles or create your own style from scratch.
Access advanced options for further control over every available aspect of your map style including visibility, fills & stroke weight.
Use the styling wizard for point and click custom style creation. |
Show what’s important, hide the rest
Custom map styling provides you with ways to tailor your map for a particular use case. Got your own transit stops and want to turn the Google ones off? We’ve got you covered. Want to hide highways and highlight water features? Done. You can control the visibility of labels, landscapes, transit icons, points of interest, roads and more to create the look that reflects your brand and purpose. See the samples for Android, iOS and JavaScript.
Both the iOS and the Android SDKs now support business points of interest as well; this means you’ll now see hotels, restaurants and shops on your maps. They’ll only be visible when you compile with the latest SDKs and you can control their visibility via styling.
Style once, use on any platform
When you’re happy with your new map style, you can export & use the same JSON styling object in our iOS, Android and JavaScript Maps APIs. The Styling Wizard also provides the URL for you to use with the Google Static Maps API.
To enable a custom style in your app or website, take a look at the code samples: Android, iOS & JavaScript.
You can distribute the styles with your app, fetch them dynamically, and even change them at runtime.
Custom styles now work on native iOS and Android apps as well as the web. |
The Android and iOS release notes contain details of bugs fixed as well as the custom basemap styling features mentioned in this post. Read the Maps APIs styling guides for Android, iOS and JavaScript, and watch the Styling your Maps Geocast (embedded below).
A big thank you to Android and iOS developers everywhere for using the Google Maps Android API and the Google Maps SDK for iOS and submitting feedback via the issue tracker. We heard you!
Share your styled basemaps on Twitter and G+ via #mapstyle and show us what you’ve done!
Posted by Megan Boundey, Product Manager, Google Maps Mobile APIs |
Making the most of the Google Maps Web Service APIs
September 15th, 2016 | by Maps Devel | published in Google Maps
When it comes to app development, there can be a disconnect between the robust app we intended to build and the code we actually get into a minimum viable product. These shortcuts end up causing error conditions once under load in production.
The Google Maps API team maintains client libraries that give you the power to develop with the confidence that your app will scale smoothly. We provide client libraries for Python, Java, and Go, which are used by thousands of developers around the world. We’re excited to announce the recent addition of Node.js to the client library family.
When building mobile applications, it is a best practice to use native APIS like Places API for Android and Places API for iOS where you can, but when you find that your use case requires data that is only available via the Google Maps APIs Web Services, such as Elevation, then using these client libraries is the best way forward.
These libraries help you implement API request best practices such as:
- Requests are sent at the default rate limit for each web service, but of course this is configurable.
- The client libraries will automatically retry any request if the API sends a 5xx error. Retries use exponential back-off, which helps in the event of intermittent failures.
- The client libraries make it easy to authenticate with your freely available API Key. Google Maps APIs Premium Plan customers can alternatively use their client ID and secret.
- The Java and Go libraries return native objects for each of the API responses. The Python and Node.js libraries return the structure as it is received from the API.
The client libraries can help you in a variety of ways. One of them is exposing the result sets in a format that makes most sense for the language in question. For example, the Java and Go client libraries include object hierarchies that are type-safe representations of the potential results for each API. This allows you to write code in the comfort of your editor with the knowledge that the compiler will catch any mistakes.
With 3 million apps and websites using Google Maps APIs, we have an important tip for ensuring reliability when using web services: call APIs from a server rather than directly from Android or iOS. This secures your API key so that your quota can’t be consumed by a bad actor, along with being able to add caching to handle common requests quickly.
A server instance acts as a proxy that takes requests from your Android and iOS apps and then forwards them to the Google Maps Web Service APIs on your app’s behalf. The easiest way to create a server side proxy is using the Google Maps Web Service client libraries from Google App Engine instances. For more detail, please watch Laurence Moroney’s Google I/O 2016 session “Building geo services that scale”.
You can learn more about the Google Maps API web services in our documentation. The easiest way to use these APIs and follow best practices is to use the Client Libraries for Google Maps Web Services. Download the client libraries for Java, Python, Go or Node.js from Github to start using them today!
|
Posted by Brett Morgan, Developer Programs Engineer |
New JavaScript Street View renderer brings rendering improvements and better mobile support
August 17th, 2016 | by Maps Devel | published in Google Maps
Street View is one of Google Maps’ most loved features, providing users with a way to explore and experience the world around them. Developers all over the world use Street View in the Google Maps JavaScript API to make their apps more unique and exciting, giving their users a sense of what it’s like to visit a place in real life.
Today we’re making Street View even better, especially on mobile devices, by launching a new Street View renderer in the Google Maps JavaScript API. Read on for the full details of what we’ve improved!
Better display
Smoother Transitions
Transitions from one point to another in Street View now include more animation frames, creating the effect of gliding smoothly to the next location. Transitions in the old renderer looked like jumping from one location to another.
Old renderer
|
New renderer
|
Smoother Loading Animations
The old renderer repeats images while loading new content, resulting in a stuttering effect. The new renderer uses lower resolution imagery while loading, resulting in a smoother animation when rotating an image in Street View.
Old renderer
|
New renderer
|
|
|
Object modeling improvements
Objects in Street View look better in the new renderer because it builds a 360-degree model that considers all possible perspectives. For example, this high rise building has wavy lines in the old renderer, as opposed to crisp lines in the new renderer.
Old renderer
|
New renderer
|
In another example: for imagery on an incline, such as a street with a steep hill, the new renderer corrects the objects to be vertical, whereas the old renderer would have shown the objects at an angle.
Old renderer
|
New renderer
|
Better mobile support
WebGL imagery
The new renderer uses WebGL (on browsers that support it) which results in a higher frame rate and better rendering, especially on mobile devices. On mobile devices, the old renderer would display a fish-eye projection of the image, whereas WebGL allows us to present a rendered sphere that looks as it would in reality. For example, the street in the image below is straight, but the old renderer made it look curved on mobile devices.
Old renderer
|
New renderer
|
|
|
Touch support
As mobile web usage grows, users expect familiar touch-based interactions to work everywhere. The new renderer supports the same natural touch-based gestures on mobile which have been available in the Google Maps Android app: pinch-to-zoom and double-tap-to-go. In the old renderer, zooming was only available through the +/- buttons, and movement was only possible by clicking the arrows on the ground.
Motion tracking on mobile devices
Mobile devices give developers the opportunity to provide their users with more natural ways to explore and interact with their applications. We’ve enabled support for device orientation events on Street View so that users on mobile devices can look around in Street View by moving their phone. Developers have the option to turn this off if they prefer. Please see the developer documentation for more details, or open the documentation link on a mobile device to see motion tracking in action.
Better controls
X Forward
When using a desktop device with a mouse or trackpad, users will see a small “X” at the cursor location that indicates the next camera location if they choose to move forward. Arrows indicate the direction of movement. Wall rectangles identify the direction the camera will point towards.
Next image targets
|
Next centered image target
|
|
|
Cleaner street names, labels and targets
Street names and labels are now separated from controls, removing overlap issues and allowing for clean display in right-to-left and left-to-right languages.
Old renderer
|
New renderer
|
|
|
We hope you enjoy using the new and improved Street View renderer! Also a big thank you to all the developers who use the Google Maps JavaScript API and provide feedback via the issue tracker. Getting feedback from developers is vital for us to be able to keep improving our products, so if you have any bug reports or feature requests, please let us know!
For more information on Street View in the Google Maps JavaScript API, please see the developer documentation.
|
Posted by Elena Kelareva, Product Manager, Google Maps APIs |
Keep users focused on what’s important with the latest Google Maps Android API
August 1st, 2016 | by Maps Devel | published in Google Maps
Released today, the latest version of the Google Maps Android API includes more developer requested features: you can now track camera movements more accurately via our new camera listeners, set the minimum & maximum zoom levels on your map, and restrict the user’s panning to particular lat/lng bounds of the camera target. In addition, we’ve added a new marker Tag property so you can now associate your own data object with a marker.
Track camera movements more accurately
As one of our top requests, developers have been asking for a better way to track camera movements and the ability to see why the camera is moving, whether caused by user gestures, built-in API animations or developer controlled movements [Issue 4636]. Our new camera change listeners support you in doing this. Your app can now receive notifications for camera start, ongoing, and end events.
See the developer’s guide to camera change events and take a look at this code sample which shows you how to detect when the user drags the map, and draws a line to track this movement when it happens.
Control the zooming, panning and scrolling experience
Have you ever wanted to be able to control how much your user can zoom in and out and pan around on your map so that you can more tightly control the experience? Or have you got tile overlays only for zoom levels 15 through 20 and wish you could limit the zooming capability of both the map and your tile overlays to those particular levels?
You can now set the min and max zoom levels on your map by using GoogleMap.setMinZoomPreference() and GoogleMap.setMaxZoomPreference() [Issue 4663]. These zoom levels will also apply to any tile overlays you have on your map.
In addition, you can also constrain the lat/lng center bounds of the focal point of the map (the camera target) so that users can only scroll and pan within these bounds using GoogleMap.setLatLngBoundsForCameraTarget(). This is awesome if you want your users to stay within the map area of your tile overlays, or you wish to confine the map in your app to a particular local area.
![]() |
Pan and zoom limits on a map for Adelaide, a beautiful city in South Australia
|
See the developer’s guide to learn more about setting boundaries on the map as well as this code sample.
Marker tags
Does your app cater for different types of markers and you want to treat them differently when a user taps on them? Or do you want to assign priorities to your markers? The new marker Tag property allows you to associate whatever data object you like with a marker, supporting you in doing this and more [Issue 4650].
A big thank you to Android developers everywhere for using the Google Maps Android API and submitting feedback via the issue tracker.
Our release notes contain details of bugs fixed as well as the features mentioned in this post. Take a look and start using our new features today!
Posted by Megan Boundey, Product Manager, Google Maps Mobile APIs |
Google Places API for iOS & Google Maps SDK for iOS are now in separate CocoaPods
July 25th, 2016 | by Maps Devel | published in Google Maps
In today’s release, the Google Places API for iOS 2.0 and the Google Maps SDK for iOS 2.0 are now in separate CocoaPods. For developers who only use the Google Places API for iOS, this will significantly reduce the binary size of their app.
What does this mean for me? What do I have to do?
Nothing immediately for your current implementation, but we strongly suggest that you upgrade within the next year to the new Google Maps SDK for iOS 2.0 and Google Places API for iOS 2.0. The Google Maps for iOS SDK Version 1.x will become unsupported in one year’s time.
If you are using the Standard Plan Google Maps SDK for iOS 1.x, and haven’t specified a version in your podfile, you will be automatically upgraded to the new Google Maps SDK for iOS 2.0 when you run ‘pod update’. If you use any Places functionality, we’ve created this migration guide for the Places API to step you through the process of migrating to the new Google Places API for iOS 2.0.
In addition, we’ve documented how to extract all the frameworks (Maps, Places) from the relevant CocoaPods so you can manually include the SDKs in your project rather than using CocoaPods if you wish. [Issue 8856]
What does this mean for Premium Plan Maps SDK customers?
There is no longer a separate Google Maps Premium Plan SDK. Instead it has been replaced with the new streamlined Google Maps SDK for iOS 2.0 for both Standard and Premium Plan developers.
We’ve created a Premium Plan migration guide that will step you through the process of migrating to the new Google Maps SDK for iOS 2.0. We’ve also documented how to extract the frameworks from the CocoaPods so you can manually include the SDKs in your project if you’d prefer that. Your Enterprise Maps key will continue to work, as will your Premium Plan.
Please note:
The Google Maps SDK for iOS Premium Plan SDK 1.13.2 (current version) will be supported for one year during which time we suggest you upgrade to the new streamlined Google Maps SDK for iOS 2.0.
Take a look at our release notes and start using version 2.0 today!
|
Posted by Megan Boundey, Product Manager, Google Maps Mobile APIs |
I/O session: Location and Proximity Superpowers: Eddystone + Google Beacon Platform
July 25th, 2016 | by Maps Devel | published in Google Maps
Bluetooth beacons mark important places and objects in a way that your phone understands. Last year, we introduced the Google beacon platform including Eddystone, Nearby Messages and the Proximity Beacon API that helps developers build beacon-powered proximity and location features in their apps.
Since then, we’ve learned that when deployment of physical infrastructure is involved, it’s important to get the best possible value from your investment. That’s why the Google beacon platform works differently from the traditional approach.
We don’t think of beacons as only pointing to a single feature in an app, or a single web resource. Instead, the Google beacon platform enables extensible location infrastructure that you can manage through your Google Developer project and reuse many times. Each beacon can take part in several different interactions: through your app, through other developers’ apps, through Google services, and the web. All of this functionality works transparently across Eddystone-UID and Eddystone-EID — because using our APIs means you never have to think about monitoring for the individual bytes that a beacon is broadcasting.
For example, we’re excited that the City of Amsterdam has adopted Eddystone and the newly released publicly visible namespace feature for the foundation of their open beacon network. Or, through Nearby Notifications, Eddystone and the Google beacon platform enable explorers of the BFG Dream Jar Trail to discover cloud-updateable content in Dream Jars across London.
To make getting started as easy as possible we’ve provided a set of tools to help developers, including links to beacon manufacturers that can help you with Eddystone, Beacon Tools (for Android and iOS), the Beacon Dashboard, a codelab and of course our documentation. And, if you were not able to attend Google I/O in person this year, you can watch my session, Location and Proximity Superpowers: Eddystone + Google Beacon Platform:
We can’t wait to see what you build!
|
About Peter: I am a Product Manager for the Google beacon platform, including the open beacon format Eddystone, and Google’s cloud services that integrate beacon technology with first and third party apps. When I’m not working at Google I enjoy taking my dog, Oscar, for walks on Hampstead Heath. |
Announcing marker clustering in the Google Maps SDK for iOS Utility Library
July 18th, 2016 | by Maps Devel | published in Google Maps
Today we’ve added marker clustering to the Google Maps SDK for iOS Utility Library! This much-requested feature is now available for iOS in addition to Android and JavaScript.
Do you ever feel that your map just has too many markers on it, making it feel cluttered and hard to comprehend? Or, perhaps you want to show where the popular restaurants are in your city, but you still want your map to look clean?
Marker clustering supports you in doing this. As the zoom levels of the map change, you can aggregate markers, indicating clearly to your users exactly where those popular restaurants are located. As your user zooms in, the markers progressively split out until all of the individual markers are displayed.
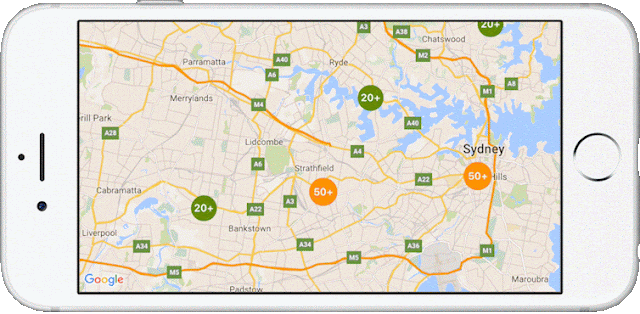
Using the new marker clustering feature in the Google Maps SDK for iOS Utility Library is an easy 4 step process:
- Add ‘Google-Maps-iOS-Utils’ to your Podfile
- Instantiate the GMUClusterManager
- Implement the GMUClusterItem protocol for your marker objects
- Add the marker objects to the cluster manager
We provide the default algorithm, renderer and icon generator to support you in doing this. In addition, you can also fully customize each of these by extending the default implementations, or by providing your own implementation of these protocols: GMUClusterAlgorithm, GMUClusterRenderer, GMUClusterIconGenerator.
Take a look at the documentation and demo samples, and start using marker clustering in the Google Maps SDK for iOS Utility Library today!
|
Posted by Megan Boundey, Product Manager, Google Maps Mobile APIs |
I/O session live: Building geo services that scale
July 14th, 2016 | by Maps Devel | published in Google Maps
While at Google I/O, l had the opportunity to present the session ‘Building geo services that scale’. I’m pleased to share it more broadly for those of you who were not able to be there in person:
Building geo services that scale
Not all map and geo applications run entirely on your mobile device. Perhaps you want to protect your keys or other API access data from reverse engineering by putting them in the cloud, or you have custom business logic that you run on your server that you don’t want to distribute via mobile. To protect your keys and API access data you’ll need to operate some kind of service. In this session you’ll learn how to build that service on the Google Cloud Platform and consume it in a mobile application that uses the Google Maps APIs.
|
About Laurence: I am a Developer Advocate at Google, working on mobile services, specializing in cross-platform developer technologies. As the host of ‘Coffee with a Googler’ on the Google Developers channel I’m able to meet with some of those most creative and inspiring developers at Google and learn about the projects they’re leading. When I’m not Googling, I’m involved in many things, including working on the revival comics for the Stargate TV shows, and enjoying the geek cred that this brings. |
Behind the Australian Elections Map: Mapping democracy in real-time
July 1st, 2016 | by Maps Devel | published in Google Maps
It’s elections time in Australia! With 94% of eligible Australians registered to vote, there will be close to 15 million participants this year.
Googlers in the Sydney office were recently chatting about the upcoming election and realized we all had similar questions: what were the results last cycle, where are the closest polling stations, and where do we look for real-time results on election day? Friends and family were asking the same questions, so we decided to build a solution using Google Maps APIs, Firebase, Google App Engine, Google Cloud Compute, Angular 2, the Google Translation Toolkit and Go.
The aim of the election map was to provide all the information that Australians would need to participate in the voting process. We wanted to cover pre-, mid- and post-election needs, including:
- A polling place locator with searchable addresses, suburbs, and electorates
- Directions and navigation to the polling places, accessible via the election map
- Real-time election results once polling has closed and counting has started
- The ability to share and embed maps.
![]() |
UX mockup: map and fake election results using testing data |
The pre-election map displays static electorate data, polling booths and ballot papers. It also indicates who won the electorate in the last 2013 election. To do this, we sourced 2013 election data from the Australian Electoral Commission (AEC) and stored it in a Go application intended for Google App Engine so that it could be served to the website frontend. The AEC provided us with data about electorate boundaries and polling place locations.
The website frontend was built using Angular 2 and we’ve used several open source projects, including GDAL, mapshaper and a point clustering library. These libraries allowed us to send only the required details for a user’s viewport, while keeping data usage reasonably low and reducing visual noise.
![]() |
Polling location information with address and directions intent |
Day-of website visitors will have the ability to search for polling stations and learn about what services are available at each location (accessibility, sausage sizzle and/or cake stand). We sourced the sausage sizzle and cake stand data from Democracy Sausage and Snagvotes. We used the polling place ID to match these to the AEC polling place identifiers. We built a small Google Compute Engine app which sources the data from our sausage sizzle data sources and broadcasts it out to our live web application using Firebase Realtime Database.
![]() |
Autocomplete searches for polling locations |
To enable the search functionality, we use two different services in parallel. We first attempt to match the search query against the electorate list on the client. We also use the Google Maps Javascript API Places Library with Autocomplete to provide suggestions of what users might be searching for, including suburbs, places of interest and addresses. This gives our users the ability to select recommendations instead of having to type full queries.
Voters also need to plan their trip to the polling booths. We relied on Google Maps’ existing real-time traffic information and turn-by-turn directions to provide directions functionality.
After 6pm on election night and when votes begin to be counted, we will switch the site to show real time election results. To do this, again we are using the AEC data feed and Firebase Realtime Database.
To make it really easy for people to visualize the elections results we employed a hemicycle (half donut circle) in the left sidebar to display real-time results. We also added “share” and “embed” features so people and media can easily share information about the election.
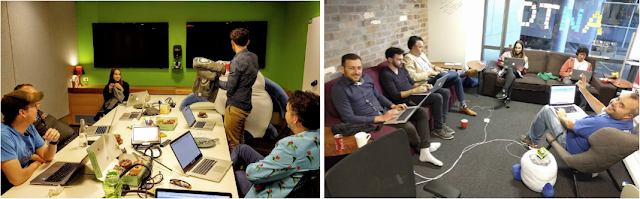
This project was put together by a cross-functional group of Google volunteers including software engineers, UX Designers, product managers and the legal and marketing teams. We’ll all be voting on July 2nd, cake in hand. See you at the polls!
![]() |
About Taylah: I am an Associate Product Manager at Google’s Sydney office where I work on Google Maps for Android Auto. I love working on Google Maps as you get to help millions of people explore the world every day. When I’m not at work, I love exploring beautiful places, shopping in thrift stores, painting and spending time with my family and friends. |